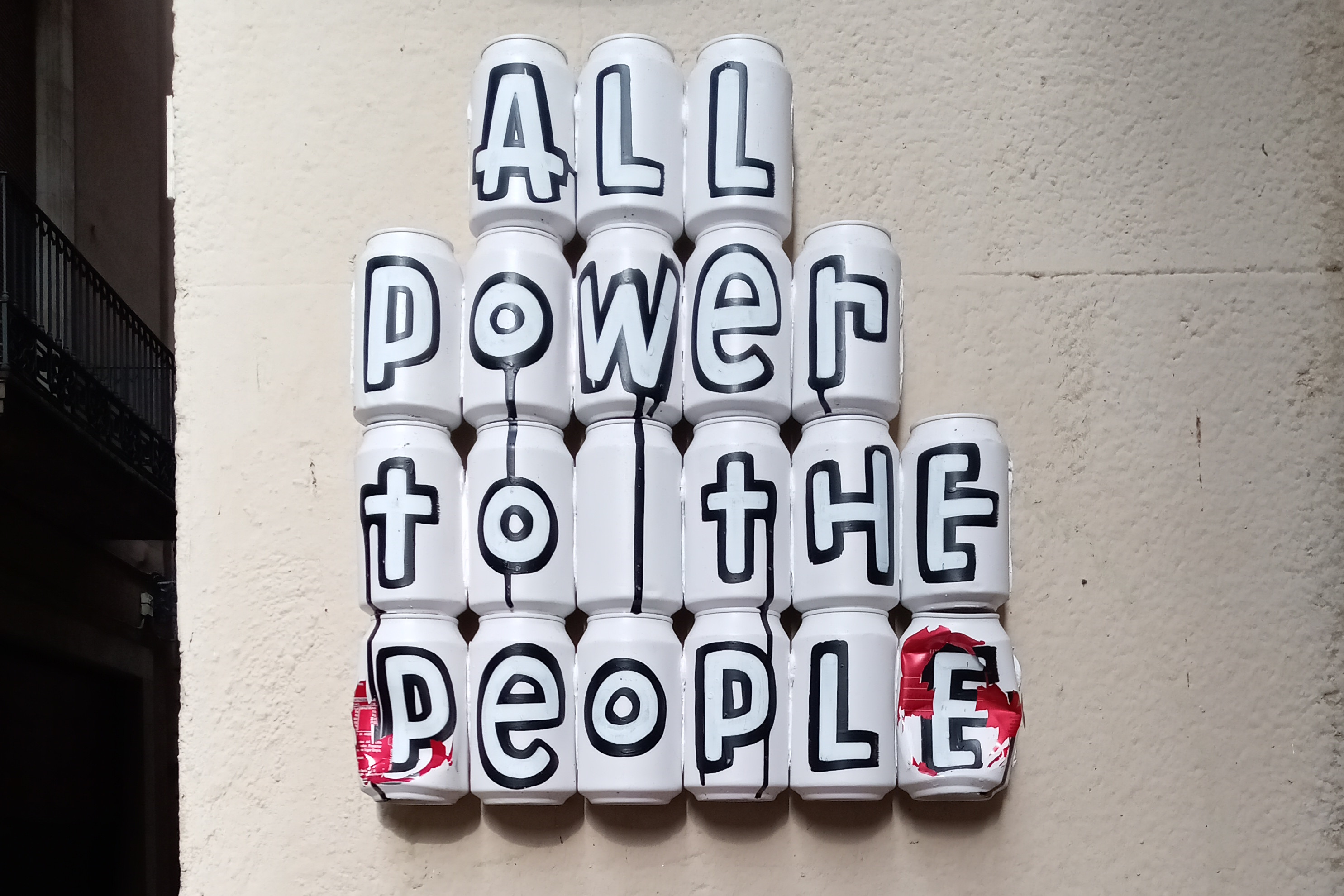
If I got a dollar for every time I’ve said that technical writers have shitty tools, I’d have dozens of dollars by now. I’ve only said that a few dozen times, but that’s still a lot. Maybe I should raise my rates. The problem isn’t the software that we use, exactly. It’s the tedious parts between the software.
Tech writers do a lot of repetitive, tedious things. Product name change? Make sure all 500 HTML pages are up to date. The spreadsheet of data centers was updated for the 3rd time this week, someone has to copy and paste the third column into the knowledge base article again.
The list goes on. Dunno about you, but sometimes I feel like I spend more time fussing and not enough time writing.
Developers have lots of repetitive, tedious things to do too. The difference between us and them? They have the skills to automate their tedious, repetitive stuff so they can code more and fuss less.
You could ask a developer to rig up some scripts for you. First, you’d have to know what to ask for. Then you’d have to bribe the developer’s manager and wait a few weeks.
Or you could write your own damned script.
Technical writers can automate their stuff too. With just a change in mindset and a bit of knowledge, you can see opportunities to free yourself and your team from tedium.
I’ve been automating workflows for years for the doc teams I’ve worked with. It saves us time and improves the quality of our deliverables. Machines should do the fussing so we can do the writing.
You can learn these skills, too. Here’s how to do it: